Bridge Pattern: Decoupling Abstraction from Implementation
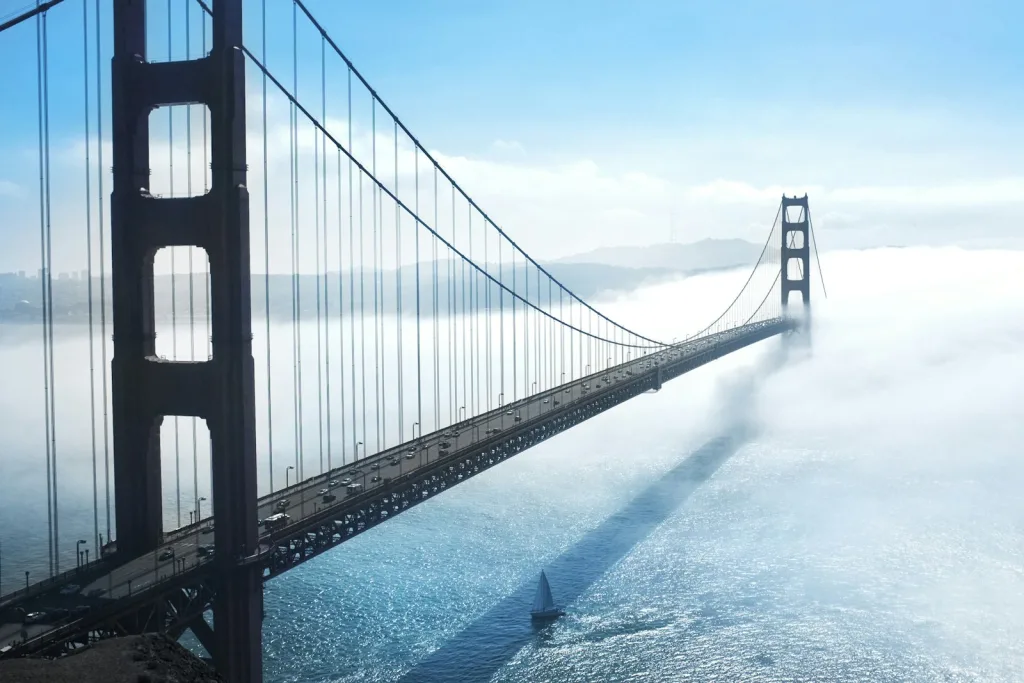
The Bridge Pattern is a structural design pattern that plays a crucial role in bridging the gap between abstraction and its implementation, allowing them to evolve independently. This design pattern is particularly useful in scenarios where software entities change frequently both in terms of their complex implementations and their abstraction layers. This guide explains the Bridge Pattern, its importance, structure, and provides practical examples of how it can be applied to enhance software design flexibility and maintainability.
Understanding the Bridge Pattern
The Bridge Pattern involves an interface which acts as a bridge between the abstraction and its implementation, making them independent of each other. This separation helps in reducing the complexity and provides a cleaner implementation of real-world software designs.
Components of the Bridge Pattern
- Abstraction: Defines the abstraction’s interface and maintains a reference to an object of the Implementor type.
- Refined Abstraction: Extends or refines the interface defined by Abstraction.
- Implementor: Defines the interface for implementation classes. This interface does not have to correspond directly to the Abstraction’s interface; in fact, the two can be entirely different. Implementor provides only primitive operations, and Abstraction defines higher-level operations based on these primitives.
- ConcreteImplementor: Implements the Implementor interface and defines its concrete implementation.
Implementation of the Bridge Pattern
Here’s a conceptual code example in Java to demonstrate the Bridge Pattern:
// Implementor
interface Device {
void turnOn();
void turnOff();
void setChannel(int channel);
}
// ConcreteImplementor
class Television implements Device {
public void turnOn() {
System.out.println("Television turning on.");
}
public void turnOff() {
System.out.println("Television turning off.");
}
public void setChannel(int channel) {
System.out.println("Television changing channel to " + channel);
}
}
// Abstraction
abstract class RemoteControl {
protected Device device;
public RemoteControl(Device device) {
this.device = device;
}
abstract void togglePower();
}
// RefinedAbstraction
class BasicRemote extends RemoteControl {
public BasicRemote(Device device) {
super(device);
}
public void togglePower() {
System.out.println("Remote power toggle requested.");
device.turnOn();
}
}
// Client
public class Client {
public static void main(String[] args) {
Device tv = new Television();
RemoteControl remote = new BasicRemote(tv);
remote.togglePower();
}
}
Benefits of Using the Bridge Pattern
- Flexibility: Allows abstraction and implementation to vary independently.
- Scalability: New abstractions and implementations can be introduced independently.
- Single Responsibility Principle: Decouples an abstraction from its implementation, allowing you to focus on high-level logic independently from the platform details.
- Reduces Class Explosion: Avoids the ‘cartesian product’ complexity explosion of implementation classes.
Practical Applications
- User Interface Frameworks: Separating a menu from its display on various devices.
- Supporting Multiple Platforms: Making software easily adaptable to different hardware platforms or systems.
- Dynamic Configuration: Allowing configurations to be changed at runtime without disrupting the system.
Conclusion
The Bridge Pattern is a powerful tool in a software developer’s toolkit, offering substantial benefits in terms of design flexibility and maintainability. It is particularly valuable in applications where both the high-level operations and their required platforms are subject to frequent changes.