Java for Newbies: Basic Concepts Explained
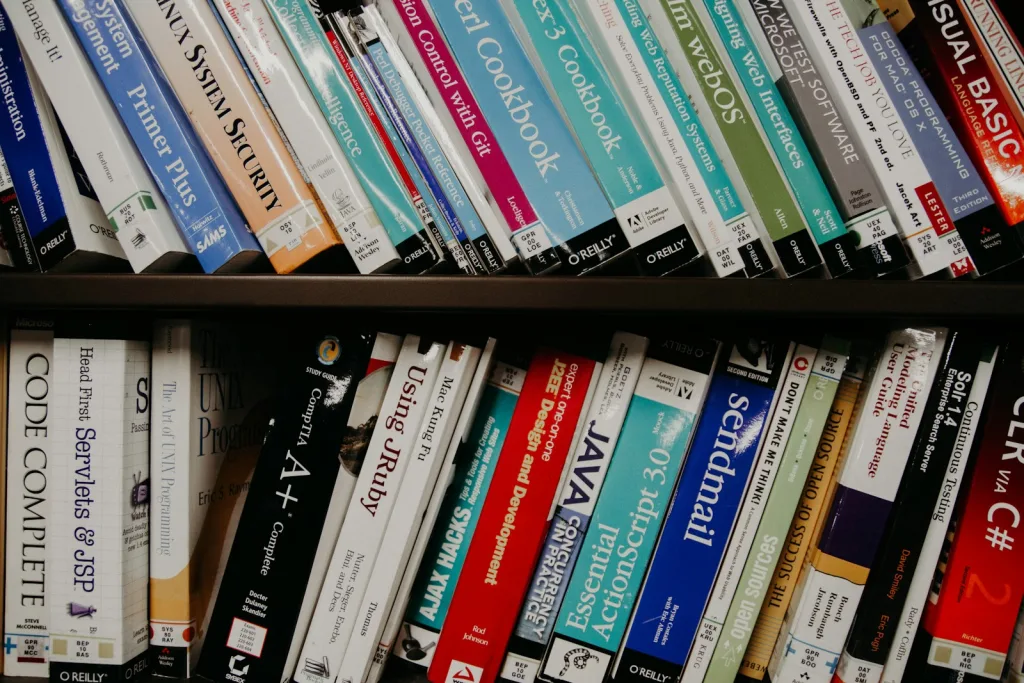
Java is one of the most popular programming languages in the world, used in everything from web applications to large systems. Ideal for beginners and seasoned developers alike, Java offers a blend of simplicity and versatility. This guide introduces the basic concepts of Java to help beginners understand how to start coding in this powerful language.
What is Java?
Java is a high-level, class-based, object-oriented programming language that is designed to have as few implementation dependencies as possible. It is a general-purpose programming language intended to let application developers write once, run anywhere (WORA), meaning that compiled Java code can run on all platforms that support Java without the need for recompilation.
Key Features of Java
- Object-Oriented: Everything in Java is associated with classes and objects, along with its attributes and methods. Key concepts include inheritance, encapsulation, polymorphism, and abstraction.
- Platform-Independent: Java code is compiled into bytecode, which can be run on any device that has a Java Virtual Machine (JVM).
- Simple and Secure: Java offers a relatively simple syntax similar to C++ but with greater emphasis on safety and security features.
- Robust and High Performance: Java is known for its reliability and efficiency, thanks to its robust memory management.
Basic Concepts in Java
- Variables: Variables are containers for storing data values. In Java, each variable must be declared with a data type.Example:
int myNum = 5; // Integer (whole number)
float myFloatNum = 5.99f; // Floating point number
char myLetter = 'D'; // Character
boolean myBool = true; // Boolean
String myText = "Hello"; // String
- Data Types: Java supports several data types, including:
- Primitive types:
byte
,short
,int
,long
,float
,double
,boolean
,char
. - Non-primitive types:
Strings
,Arrays
,Classes
, etc.
- Primitive types:
- Operators: Java provides many types of operators which include arithmetic, relational, assignment, logical, bitwise, and more.
- Control Statements:
- If-else statements allow conditional execution of code fragments.
- Loops (
for
,while
, anddo-while
) enable executing one or more lines of code repeatedly.
- Methods: Methods are blocks of code that perform a specific task. They are used to execute particular operations, to keep code reusable and organized.Example:
public static void myMethod() {
System.out.println("Hello World");
}
- Classes and Objects: Classes are templates for objects, and objects are instances of classes.Example:
public class Main {
int x = 5;
public static void main(String[] args) {
Main myObj = new Main(); System.out.println(myObj.x);
}
}
Conclusion
Java is a powerful language that is integral to modern software development. Its robust feature set and widespread support make it a great choice for new developers. By understanding these basic concepts, beginners can start their journey into the world of Java programming with confidence.