Using Observer Patterns in Event Management
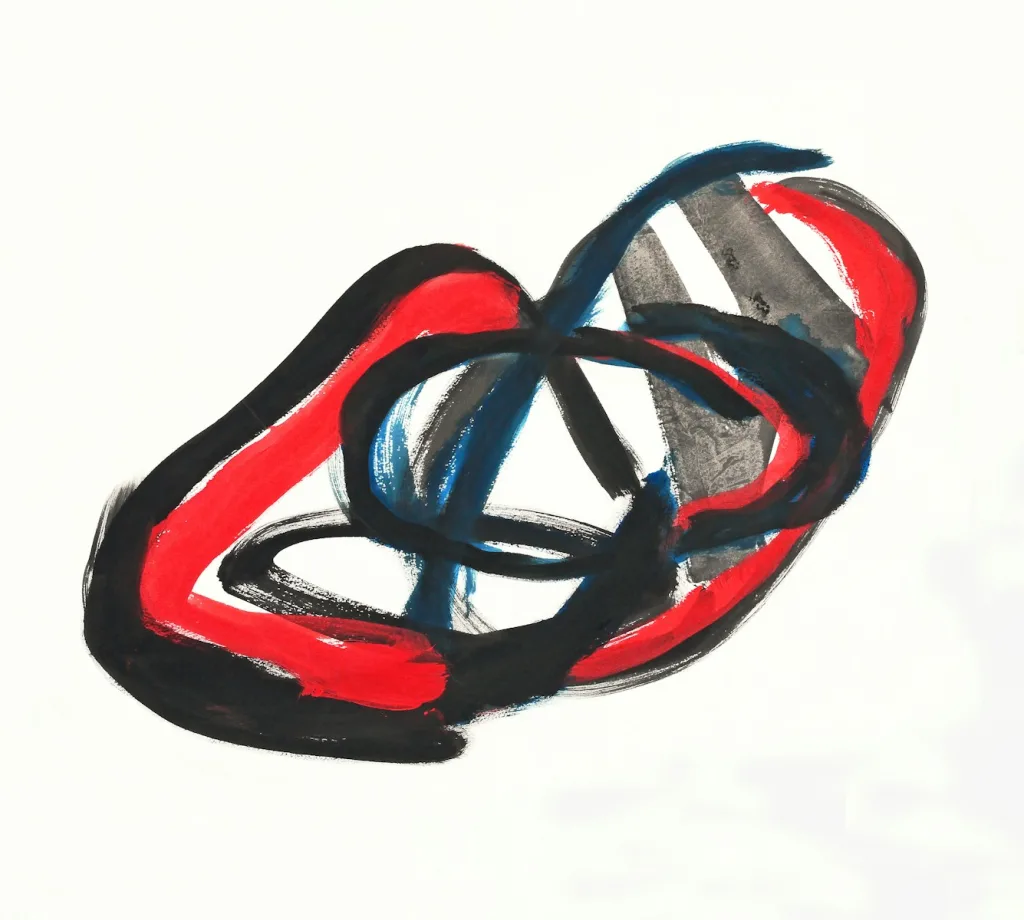
The Observer Pattern is a fundamental design pattern in software engineering, particularly useful in scenarios where an object, known as the subject, needs to notify a list of observers about changes in its state. In event management systems, this pattern is instrumental in creating a robust and flexible notification framework. This article explores how the Observer Pattern can be effectively implemented in event management, its benefits, and its real-world applications.
Understanding the Observer Pattern
The Observer Pattern involves two main components:
- Subject: The component that holds the state. When a change in state occurs, it notifies all of its observers.
- Observers: Components that are interested in updates from the subject. They react to notifications from the subject.
This pattern is particularly useful for implementing distributed event-handling systems, where one object’s state change needs to be communicated to other dependent objects without making the objects tightly coupled.
How the Observer Pattern Works in Event Management
In the context of event management, the Observer Pattern allows the event system (subject) to broadcast events to registered components (observers) such as logging, notifications, or other monitoring systems, which respond to the events without the source having to know the specifics of the responses.
Example Implementation:
Consider a simple event management system where an Event Manager notifies multiple services when an event occurs:
interface Observer {
void update(String event);
}
class EventManager {
private List<Observer> observers = new ArrayList<>();
public void subscribe(Observer observer) {
observers.add(observer);
}
public void unsubscribe(Observer observer) {
observers.remove(observer);
}
public void notifyObservers(String event) {
for (Observer observer : observers) {
observer.update(event);
}
}
}
class LoggingService implements Observer {
@Override
public void update(String event) {
System.out.println("Logging event: " + event);
}
}
class NotificationService implements Observer {
@Override
public void update(String event) {
System.out.println("Sending notification about event: " + event);
}
}
class Example {
public static void main(String[] args) {
EventManager manager = new EventManager();
Observer logger = new LoggingService();
Observer notifier = new NotificationService();
manager.subscribe(logger);
manager.subscribe(notifier);
manager.notifyObservers("New Event Launched");
}
}
Benefits of Using the Observer Pattern in Event Management
- Decoupling: The subject does not need to know anything about the observers, promoting loose coupling.
- Dynamic Subscription: Observers can subscribe or unsubscribe from the subject at runtime, allowing dynamic changes in the system.
- Scalability: New observers can easily be added as the system grows without modifying the subject.
- Reactive Programming: Supports the reactive programming model where changes propagate automatically to registered observers, ensuring consistency.
Real-World Applications
- User Interface Elements: UI components that need to react to changes in data models.
- Notification Systems: Systems where various services need real-time updates on specific events.
- Data Monitoring: Systems that monitor specific criteria and trigger actions when criteria are met.
Conclusion
The Observer Pattern is a powerful tool in the arsenal of software developers, particularly effective in managing events in complex systems. By decoupling the event-producing components from the event-consuming components, it provides a flexible and scalable solution that can handle growing system demands efficiently.